This tutorial teaches you how to access the remote desktop of CS Lab computers, so you don't have to be physically at the CS Lab to use desktop environments.
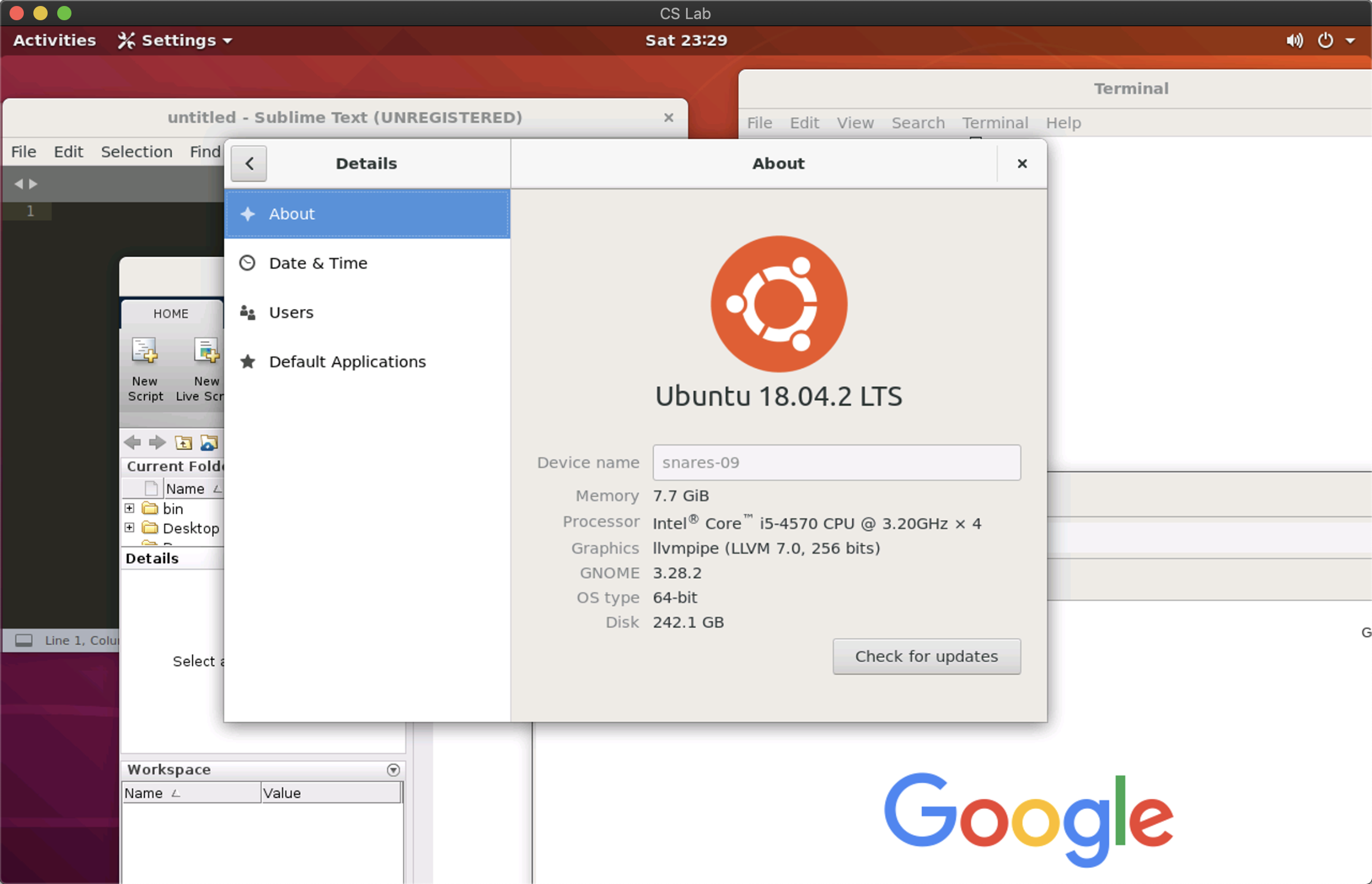
Preparation
For security reasons, the remote desktop service (port 3389) is not accessible from the public internet. You can either connect to the CS Department VPN or use
ssh
port forwarding.
To connect to the VPN, you first need to download and install
AnyConnect (not GlobalProtect) from
here. Enter
dept-ra-cssc.vpn.wisc.edu
for the server address, and choose the group to be
COMP_SCI_SPLIT
.
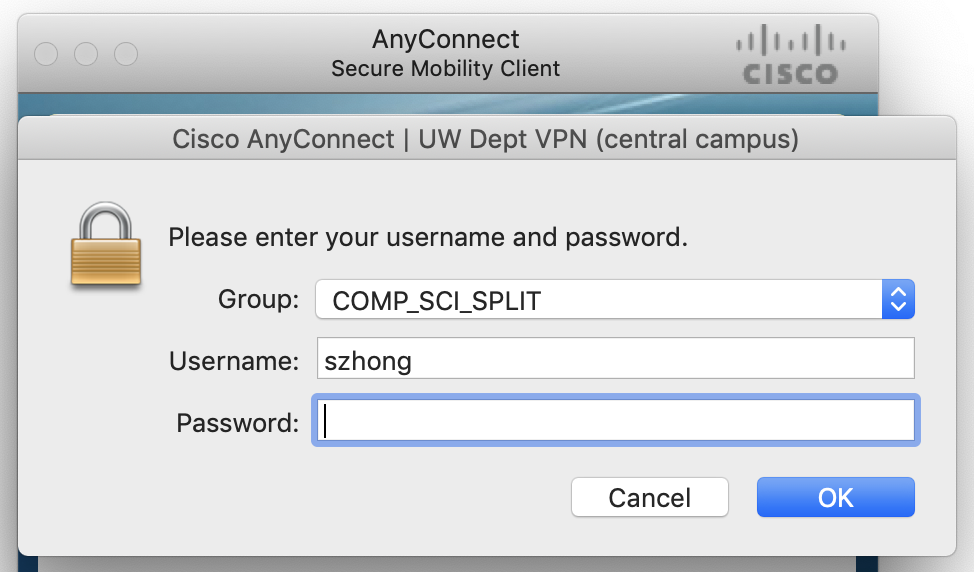
Alternatively, you can use ssh port forwarding to get access to the remote desktop service. Run the following line in your command line and enter your password.
ssh -fNT -L 3389:localhost:3389 <cs-login>@<server>
Here,
-f
stands for background,
-N
means no remote command,
-T
disables pseudo-terminal allocation and
-L 3389:localhost:3389
forwards the 3389 port at the remote server to the local computer.
You can choose
<server>
from the following list. Avoid using best-linux.cs.wisc.edu, since we don't want to connect to a different server each time.
- rockhopper-01.cs.wisc.edu through rockhopper-09.cs.wisc.edu
- royal-01.cs.wisc.edu through royal-30.cs.wisc.edu
- snares-01.cs.wisc.edu through anares-10.cs.wisc.edu
- emperor-01.cs.wisc.edu through emperor-07.cs.wisc.edu
Connect to Remote Desktop
For Windows users, press Ctrl + R to open
Run
and run the program
mstsc
. (Or navigate to Start Menu -> All Programs -> Accessories -> Remote Desktop Connection). This program should be preinstalled on all Windows machines.
Set the remote computer to be one of the CS Lab computers above (or localhost if you are using SSH port forwarding), and enter your user name.
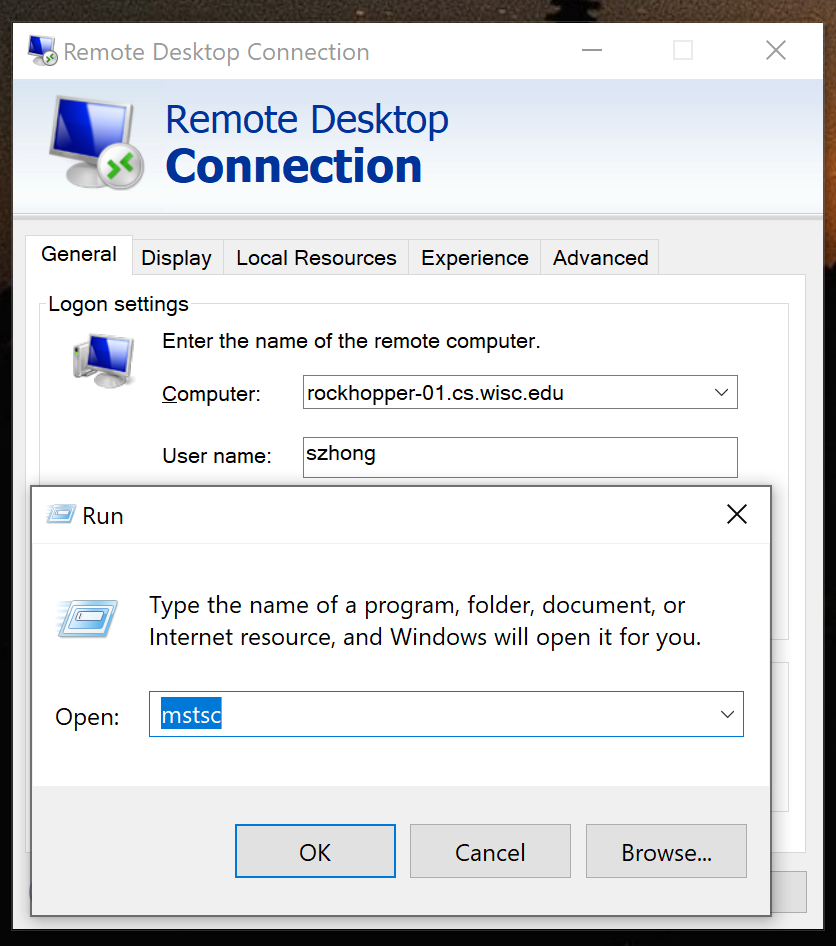
For Mac users, download Microsoft Remote Desktop from
here, and follow the configuration for Windows.
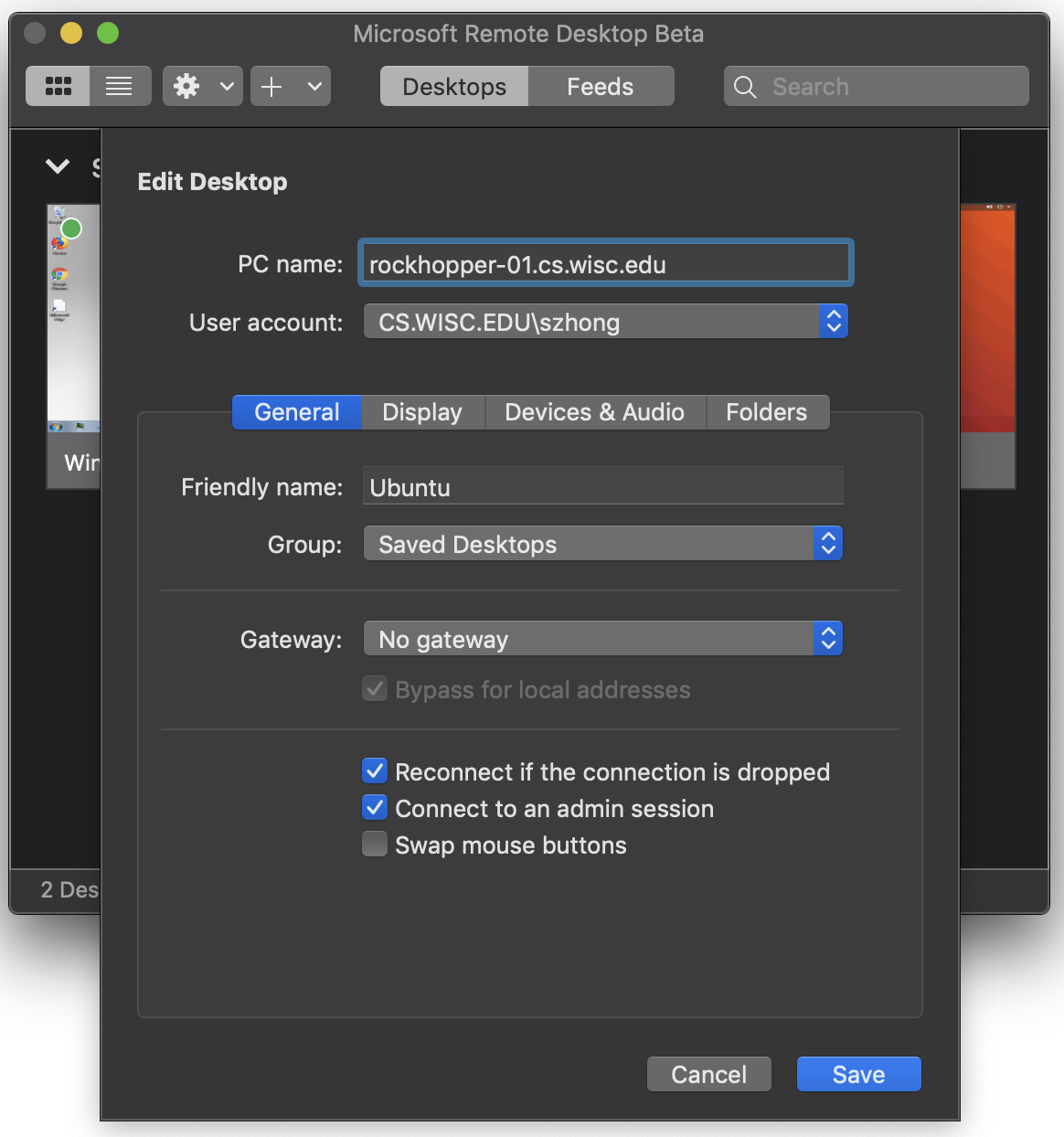
Since Microsoft Remote Desktop is also supported on Android and iOS, you can connect from your mobile devices! Here is an example at iPad with touch screen support:
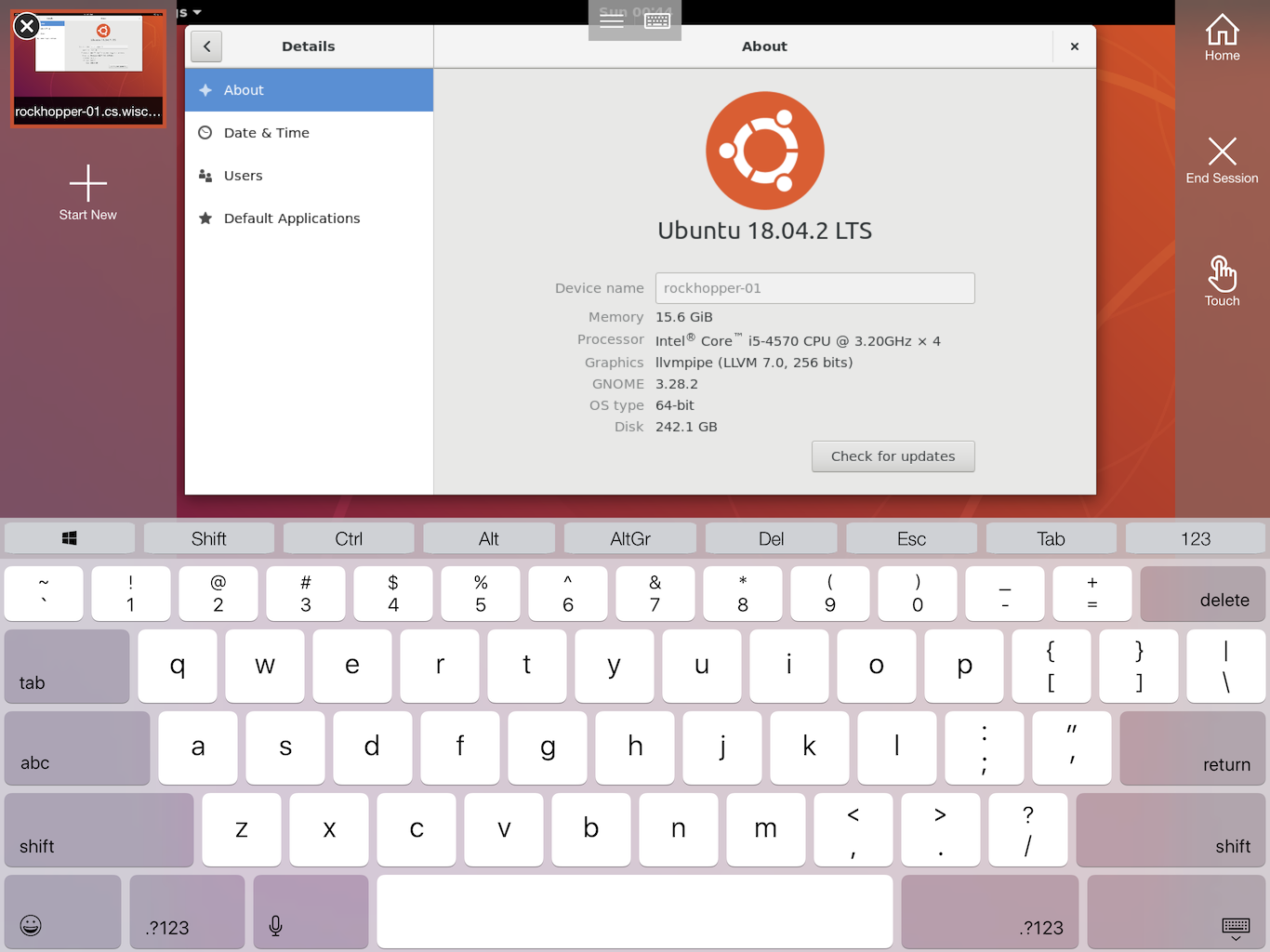
How is it useful?
There are a lot of programs pre-installed on CS Lab computers, so you don't need to configure the environment on your local computer. Examples include:
- Scientific computing: Matlab, Octave, Maple
- Data analysis: R, Jupyter Notebook, Python (with pandas, numpy, matplotlib)
- IDE & editor: VSCode, Eclipse, Atom, Sublime, Vim, EMACS
- Other: VirtualBox, TeXstudio, Slack, LibreOffice
You can start a VSCode Live Share from a CS Lab computer, and edit the code from your local computer, or share the link with your teammate for collaboration. I wrote a tutorial about
setting up Live Share and
xv6 collaborative debug. Feel free to check them out.
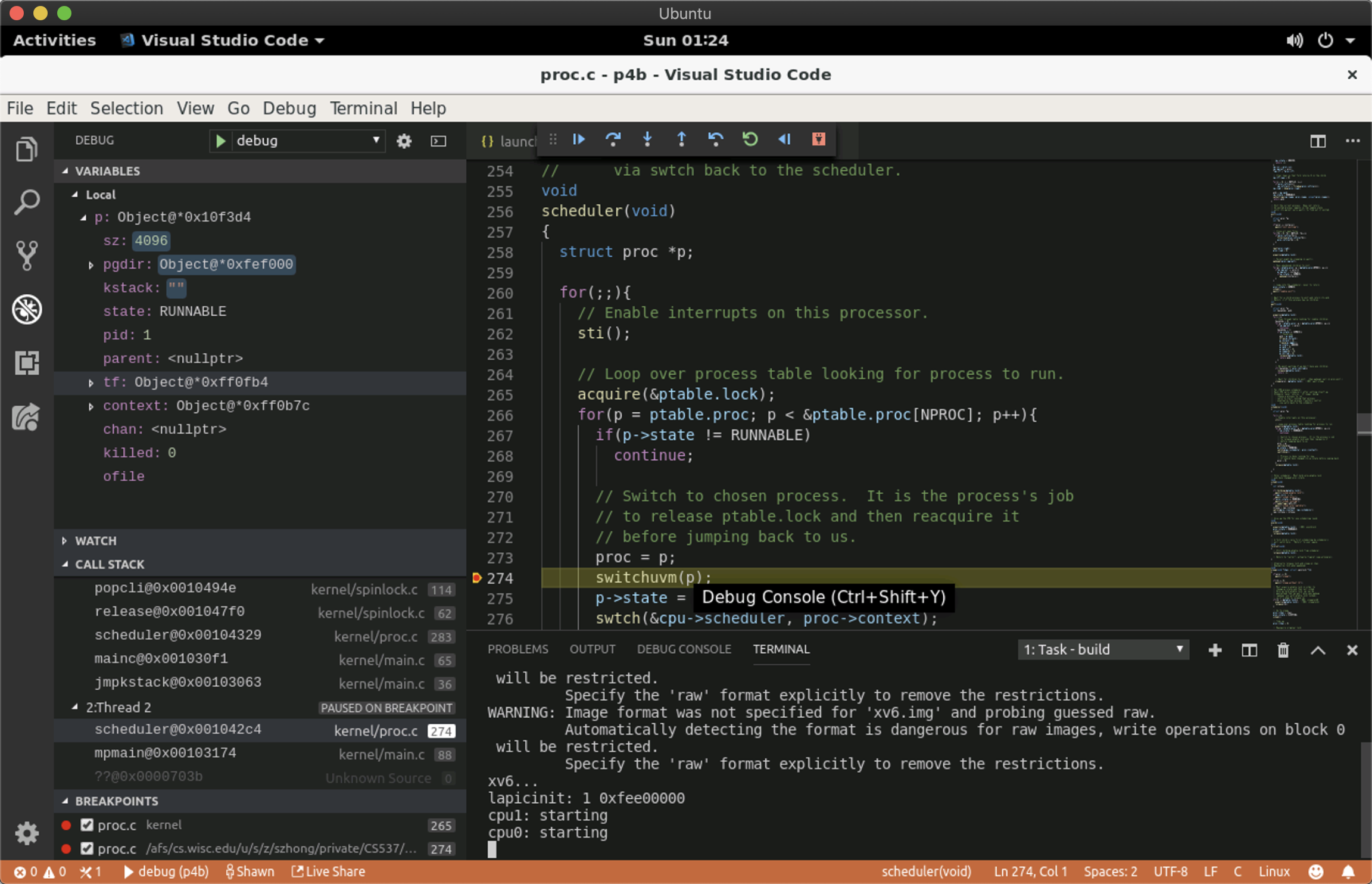
For debuging xv6 using VSCode, you need to install the Native Debug plugin, and your
launch.json
should look something like this:
{
// Use IntelliSense to learn about possible attributes.
// Hover to view descriptions of existing attributes.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"type": "gdb",
"request": "attach",
"name": "debug",
"executable": "./kernel/kernel",
"target": ":25784", // replace this with your debug port
"remote": true,
"cwd": "${workspaceRoot}",
"valuesFormatting": "parseText"
}
]
}
You can also install CLion on CS Lab computers, and work on the projects via remote desktop.
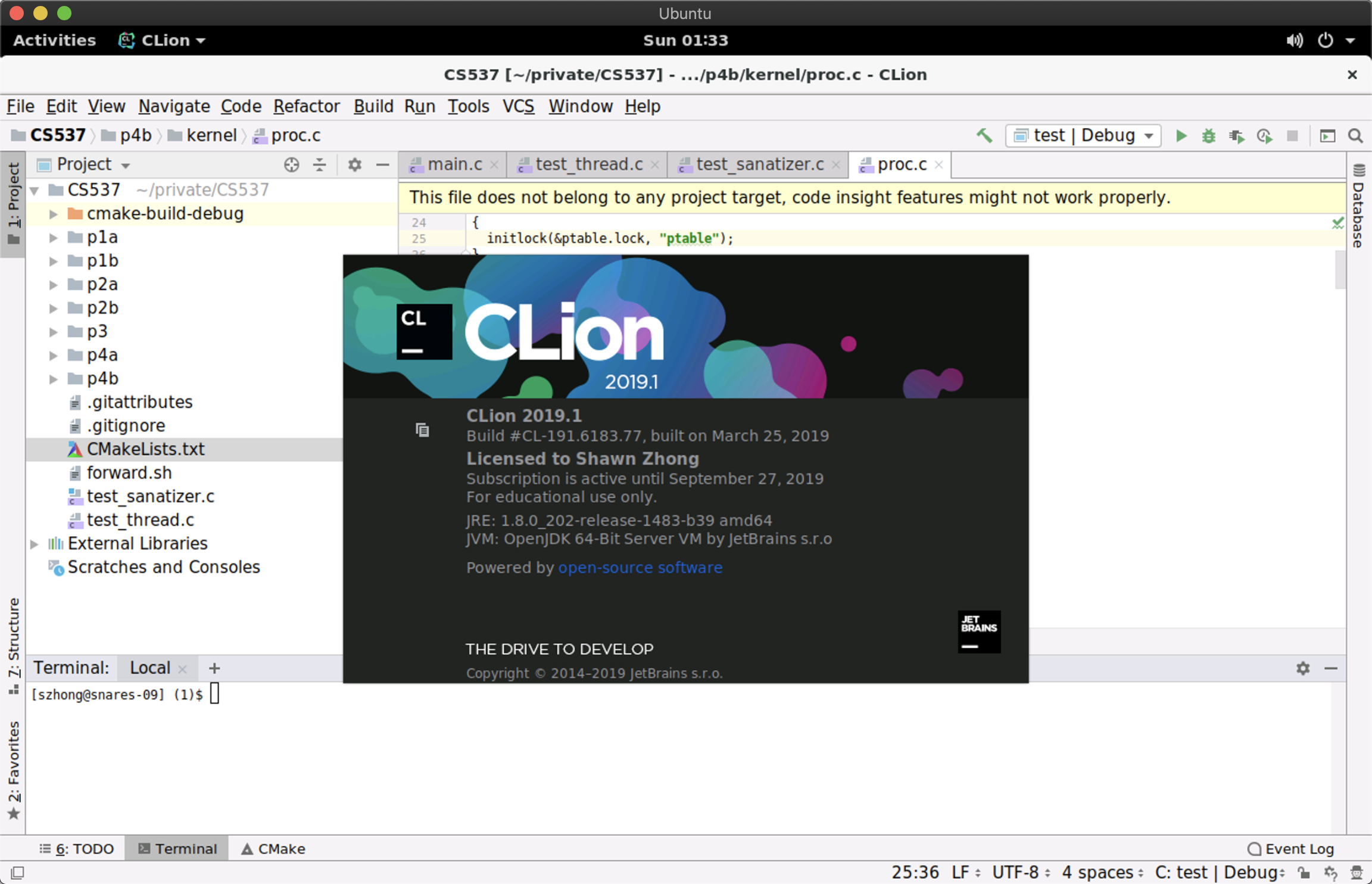
Note that your home folder (e.g.,
/u/s/z/szhong
) is mounded from a network location, so it will be relatively slow to run programs from there. One workaround is to install programs under
/nobackup
, which is stored on the local disc of the computer you connected to. To install CLion, you can download JetBrains ToolBox from
here, and set the installation folder to
/nobackup/JetBrains
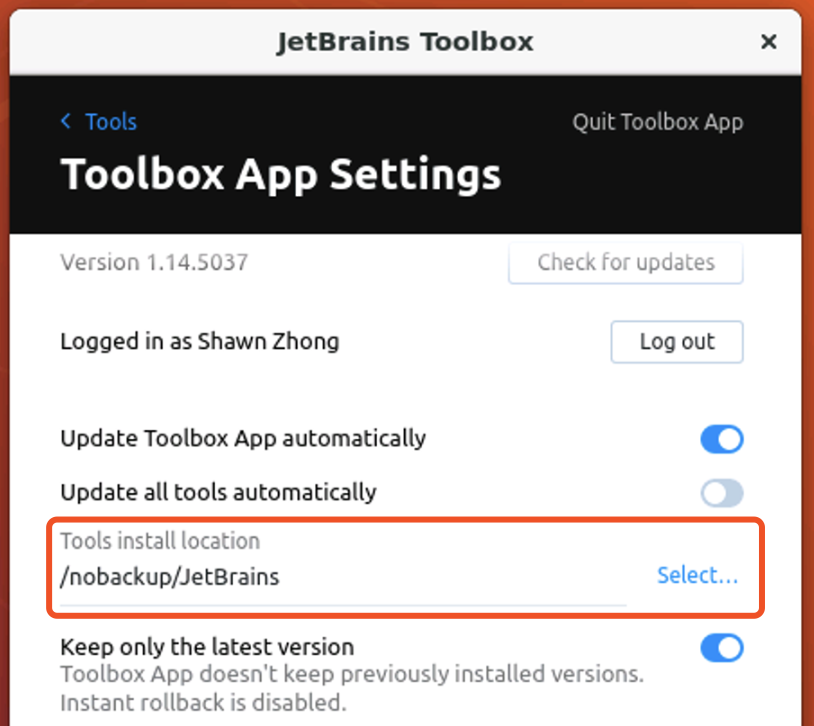
One More Thing
If you set the server address to be
rd.cs.wisc.edu
, you can connect to a Windows Remote Desktop.
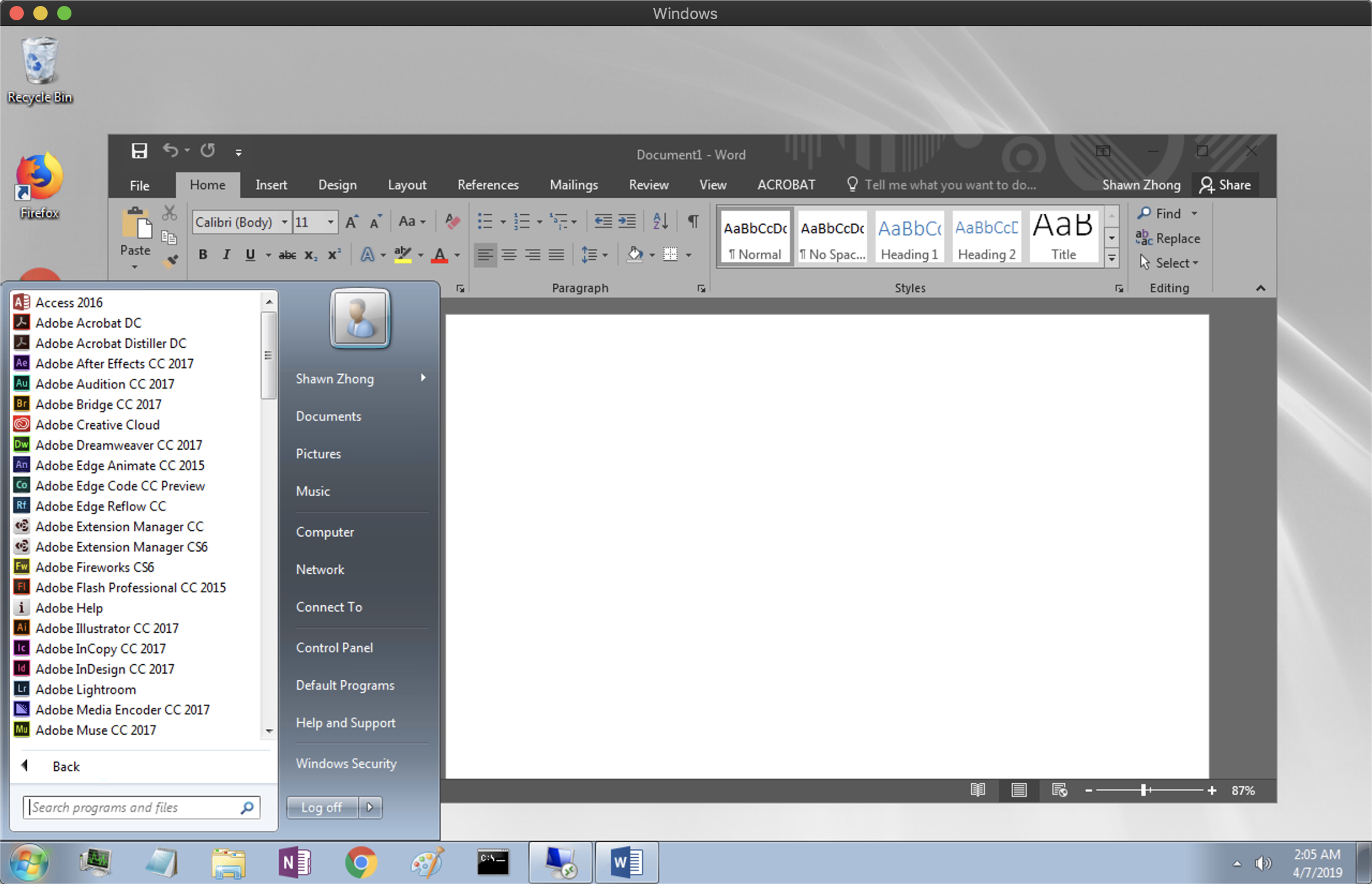
References
https://csl.cs.wisc.edu/services/remote-access/department-vpn
https://csl.cs.wisc.edu/services/remote-access/windows-remote-desktop-howto